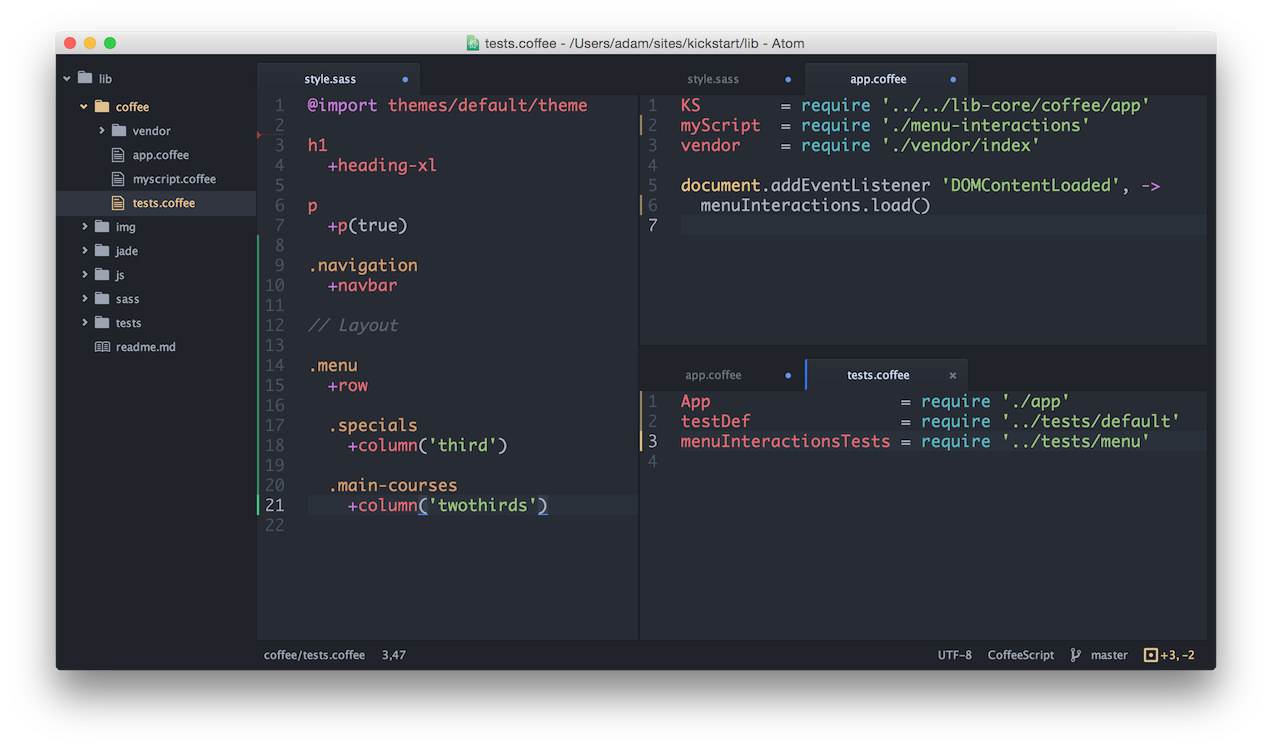
Faster and lighter than Bootstrap
CSS
Kickstart's CSS is only 80kb and even smaller when you roll your own from the Sass mixins.
JavaScript
Not only is the Kickstart JS smaller than Bootstrap and Foundation, it also doesn't require jQuery--drastically reducing page load times.